Animating Height Changes in React
Nobody likes choppy layout shifts. This article shows how to add smooth collapsing/expanding effects to animate height changes for a better user experience.
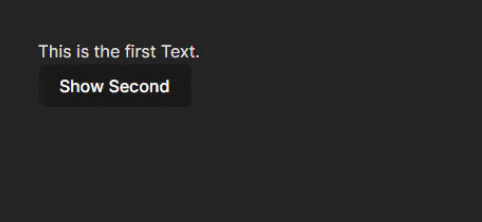
Nobody likes choppy layout shifts while interacting with a website. In this article, we will add a smooth collapsing/expanding effect to animate the height changes.
Scenario
First, let's create a height change scenario. A simple element that controls the max height of the div.
Step 1: Animate with height
We need to wrap the element with a new div, we can use this parent div to control the height via useState
.
Step 2: Observe the height change
Now that the animation is ready, we need to observe the height change of the child div. Luckily, we can use resizeObserver
. To make this happen, let's add a useRef
to reference the child div, so the resizeObserver
can access it.
After linking the childRef
and resizeObserver
, we will let the observer know when the child div resizes, set the height with setContentHeight
, the animation will do the rest!
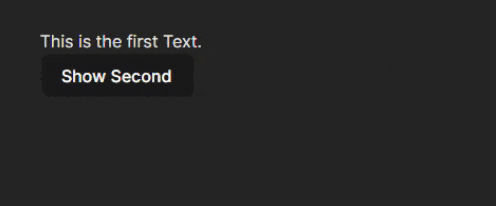
Here is the full code:
Bonus Step: Make it a component
We can always reuse code like this, so here is the extracted component:
Import and use AnimatedHeight